Certainly! Building a full-stack web application involves creating a MySQL database, connecting it with a Golang backend to query data through API services, and then displaying the data through an HTML web frontend using Vue.js. Below is a step-by-step guide for VueJS, Golang and MySQL to achieve this:
Step 1: Install MySQL
- Download and install MySQL from the official website: https://dev.mysql.com/downloads/.
- During the installation process, set a password for the root user. Make sure to remember this password.
Step 2: Install Golang and MySQL Driver
To interact with MySQL from Go, you need to use a MySQL driver. The github.com/go-sql-driver/mysql
package is a popular choice.
go get -u github.com/go-sql-driver/mysql
Step 3: Create a MySQL Database and Table
Open a MySQL command-line client or use a tool like MySQL Workbench.
Log in using the root user and the password you set during installation: mysql -u root -p
Create a new database and a table:
CREATE DATABASE exampledb;
USE exampledb;
CREATE TABLE IF NOT EXISTS users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(50) );
Step 4: Create Golang Backend
Create a new directory for your Golang project: mkdir go-mysql-vue cd go-mysql-vue
Create a file named main.go
and add the following code: package main import ( "database/sql" "fmt" "log" "net/http" _ "github.com/go-sql-driver/mysql" ) var db *sql.DB func main() { var err error db, err = sql.Open("mysql", "root:your_password@tcp(localhost:3306)/exampledb") if err != nil { log.Fatal(err) }defer db.Close() http.HandleFunc("/users", getUsers) log.Println("Server is running on :8080...") http.ListenAndServe(":8080", nil) } func getUsers(w http.ResponseWriter, r *http.Request) { rows, err := db.Query("SELECT id, name FROM users") if err != nil { log.Fatal(err) } defer rows.Close() var users []User for rows.Next() { var user User iferr := rows.Scan(&user.ID, &user.Name); err != nil { log.Fatal(err) } users = append(users, user) } // Convert users to JSON and send as the response // (You may want to use a JSON library like encoding/json here) fmt.Fprint(w, users) } type User struct { ID int `json:"id"` Name string `json:"name"` }
Replace your_password
with the MySQL root password.
Step 5: Create Vue.js Frontend
Create a new directory for your Vue.js project: mkdir frontend cd frontend
Initialize a new Vue.js project: npm init -y
Install Vue.js and Vue CLI: npm install vue npm install -g @vue/cli
Create a new Vue component. In the src
directory, create a file named App.vue
: <template> <div id="app"> <h1>User List</h1> <ul> <li v-for="user in users":key="user.id">{{ user.name }}</li> </ul> </div> </template> <script> export default {data() { return { users: [], }; }, mounted() { fetch("http://localhost:8080/users") .then((response) => response.json()) .then((data) => { this.users = data; }); }, };</script> <style> #app { font-family: Avenir, Helvetica, Arial, sans-serif; text-align: center; margin-top: 60px; } </style>
Modify src/main.js
to import and use the App.vue
component: import Vue from "vue"; import App from "./App.vue"; Vue.config.productionTip = false;new Vue({ render: (h) => h(App), }).$mount("#app");
Run the Vue.js application: npm run serve
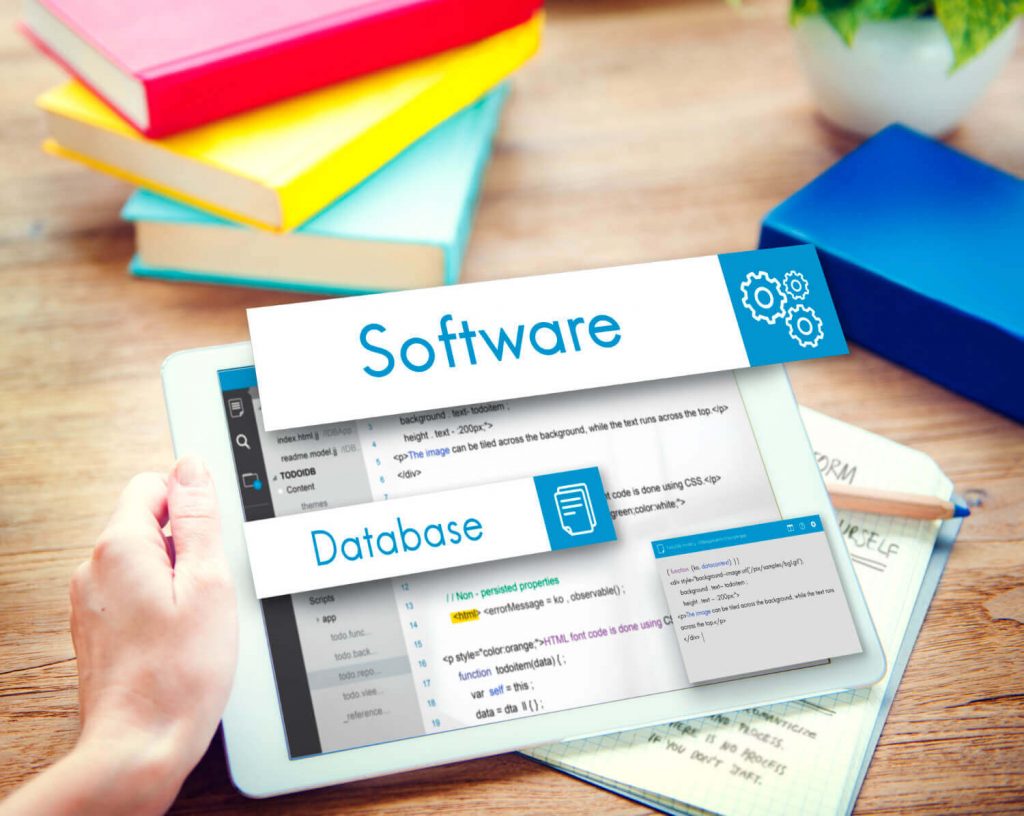
Congratulations!
Open a web browser and go to http://localhost:8080. You should see a list of users retrieved from the Golang backend. You’ve successfully created a full-stack web application with a MySQL database, a Golang backend API service, and a Vue.js frontend. This example demonstrates a simple user list, but you can expand and modify the code to suit your application’s requirements.
Cover Image : Image by DC Studio on Freepik